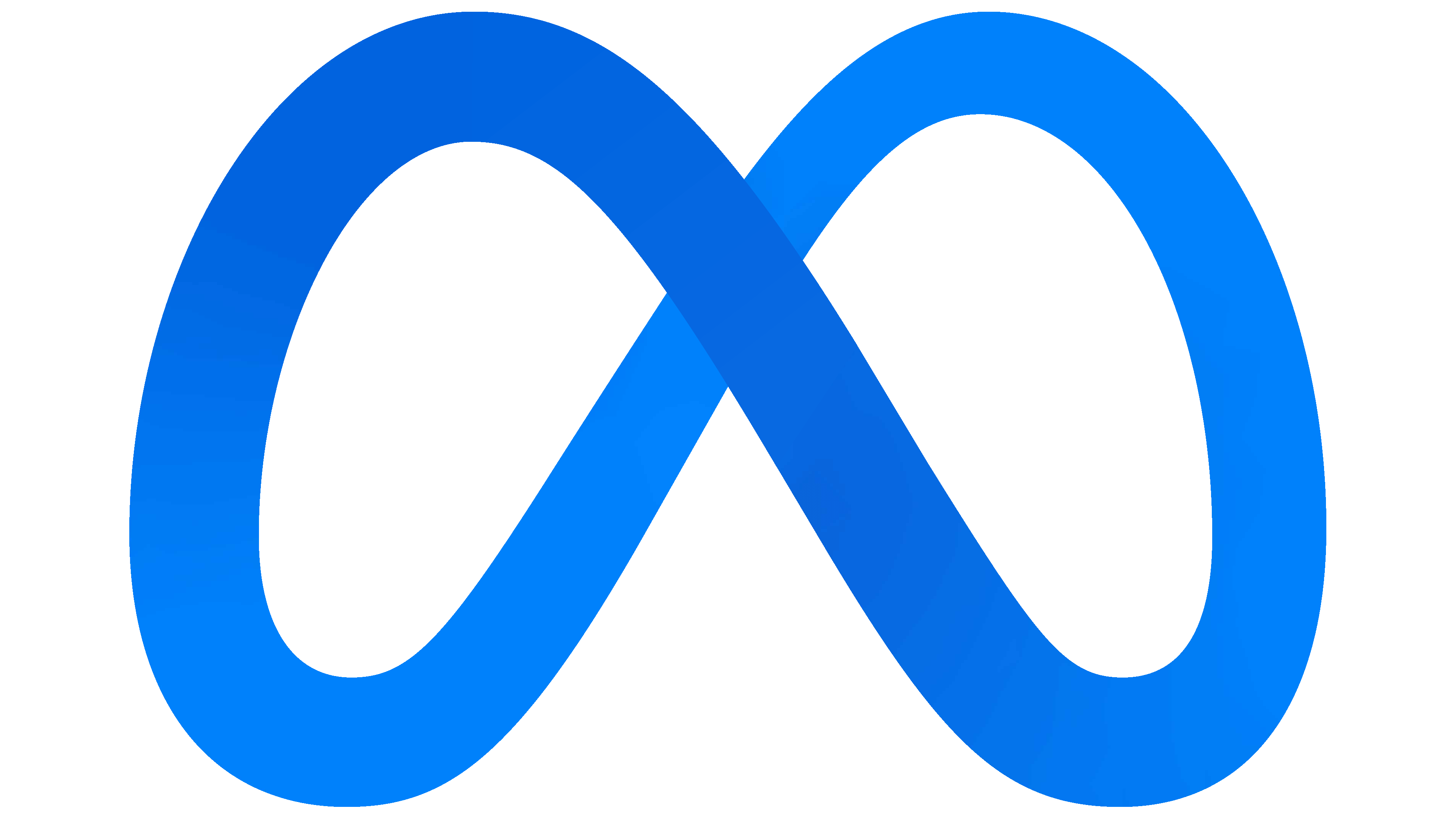

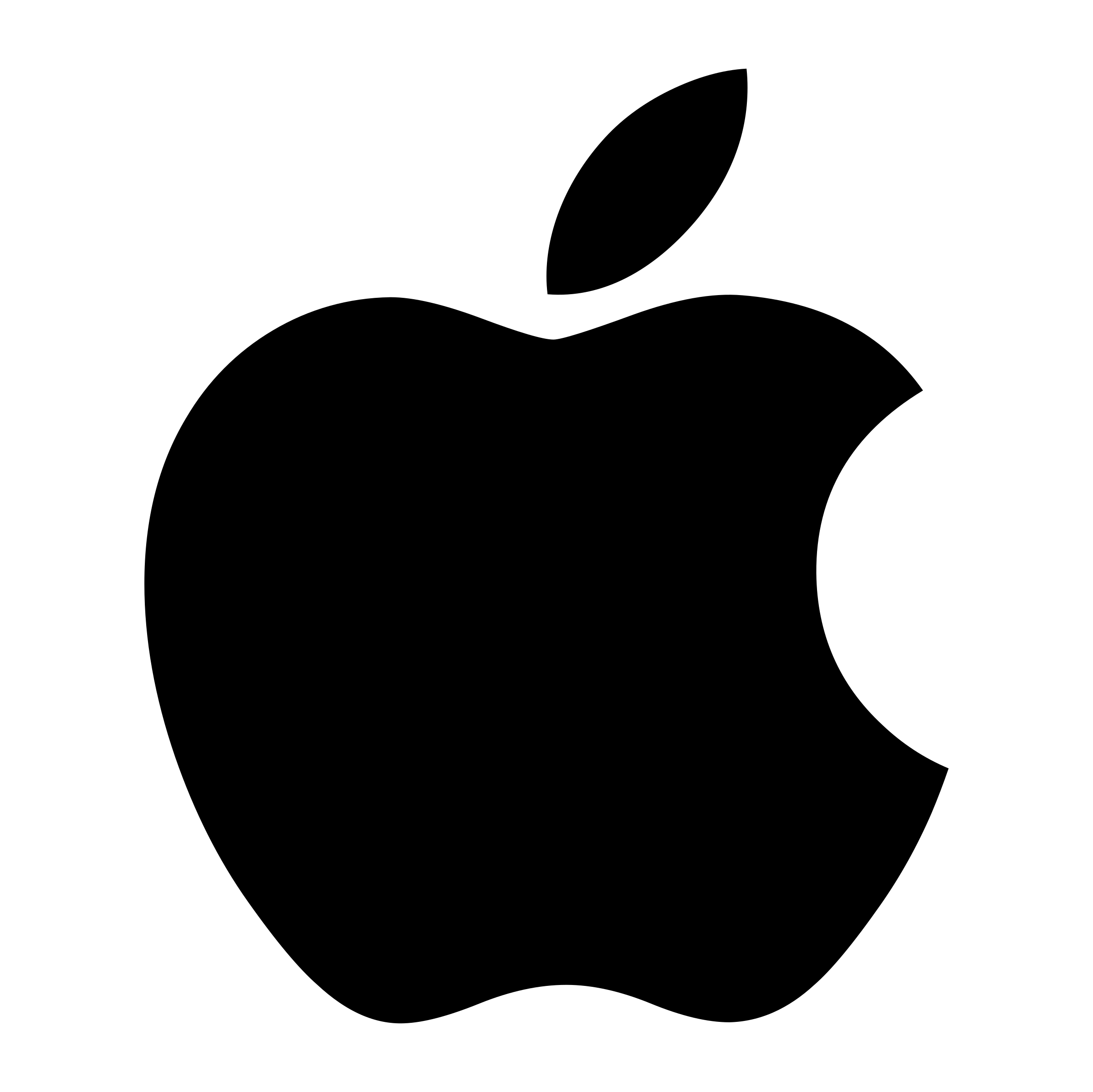
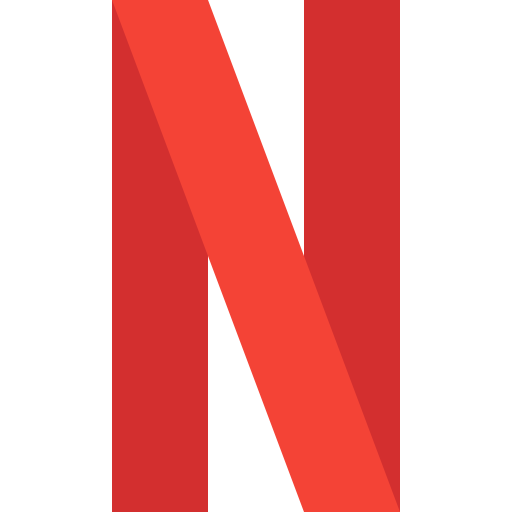
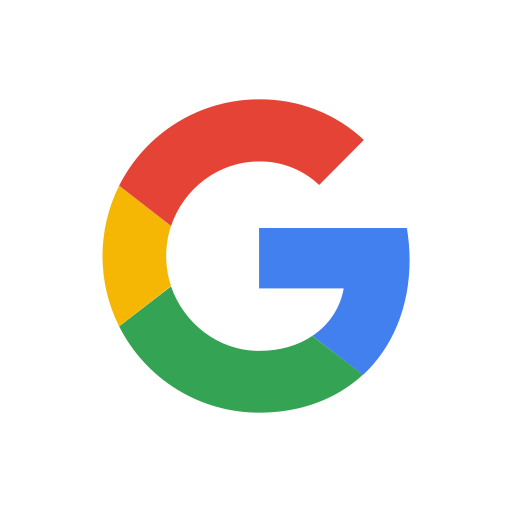
Jb Tak Fodega Nhi 🎯 Tb Tk Chodega Nhi 📚 (MAANG)
DPL34 Wildcard Matching 🔥
Given an input string (s) and a pattern (p), implement wildcard pattern matching with support for '?' and '*' where:
How To Solved this Problem
For every index of string S1, we have different options to match that index with string S2. Therefore,
we can think in terms of string matching path as we have done already in previous questions. Again
this Problem Based on the DP on String Matching
As there is no uniformity in data, there is no other way to find out than to try out all possible ways. To do so we will need to use recursion.
Recursice Approch
Steps to form the Recursive SolutionStep 1: Express the problem in terms of indexes.
Step 2: Try out all possible choices at a given index.
Now, i and j represent two characters from strings S1 and S2 respectively. There are only two options that make sense: either the characters represented by i and j match or they don’t.
Case 1: When the characters matchSIf this is true, the characters at i and j match, we can simply move to the next characters of both the strings. So we will just decrement both i and j by 1 and recursively find the answer for the remaining string portions. We return f(i-1,j-1). The following figure makes it clear.
Case 2: When the characters don’t match
- Case1: S1[i] == ‘?’
- Case2: S1[i] == ‘*’
- Case3: S1[i] is some other character
In this case, we can explicitly match ‘?’ at index i of S1 with the corresponding character at index j of S2. And then recursively call f(i-1,j-1) to check for the remaining string.
Case 2: If S1[i] == ‘*’
This is an interesting case as now ‘*’ can be replaced with any sequence of characters( of length 0 or
more) from S2.
We will revisit this example:
If any of these cases return true, we can say that the characters do match. The next question is
how to try all possible ways?
We are using two pointers i and j to represent characters of strings S1 and S2. We can surely write a
for loop to compare characters from 0 to j of S2 for the above scenario. Can we do it more
smartly? Yes, we can. Please understand the approach explained below.
We are using a recursive function f(i,j). If we do only the following two recursive calls:
The following recursive tree will help us to understand the recursion better.
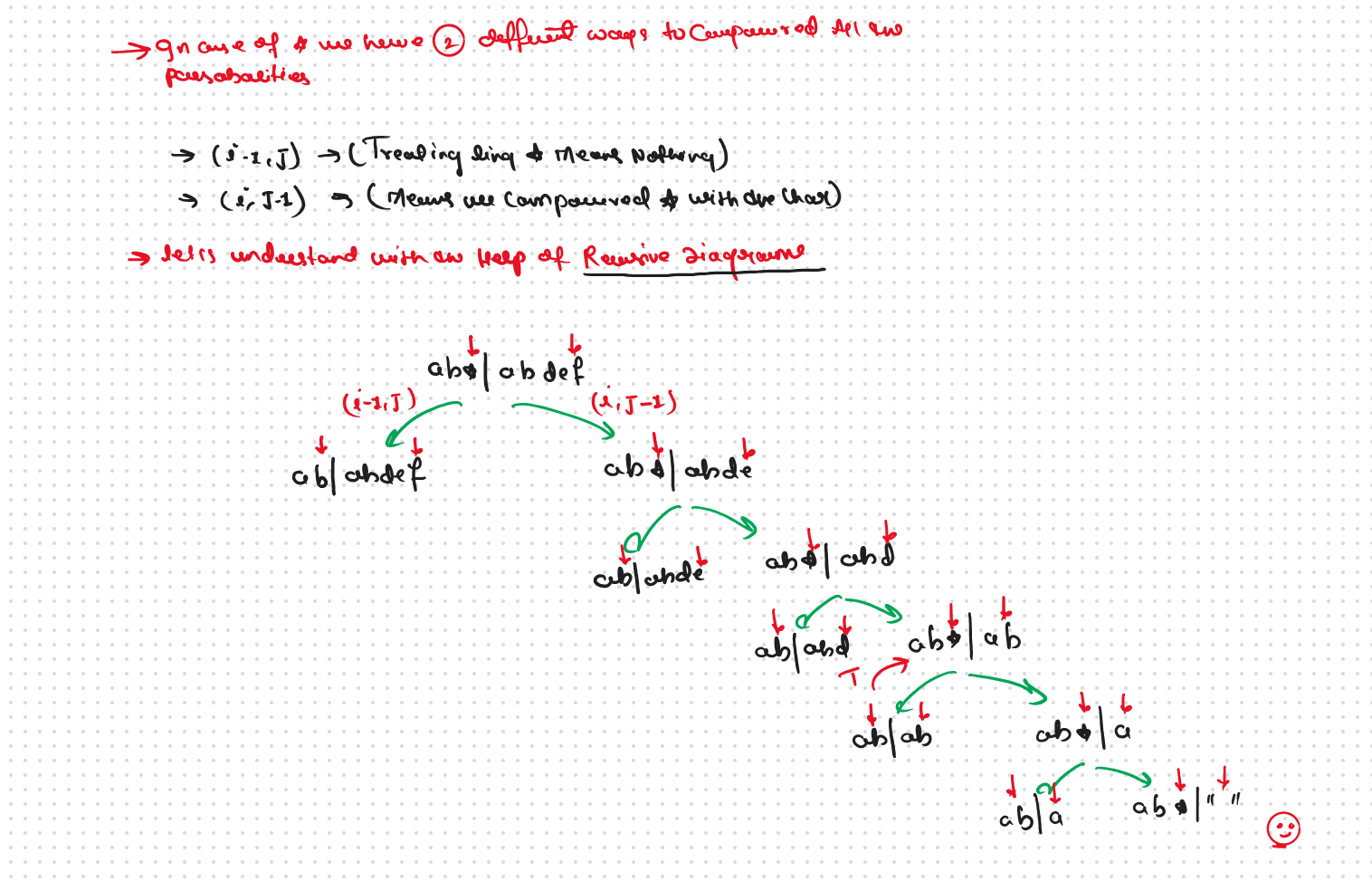
So we see how we can tackle all the required cases associated with ‘*’ by using recursion. Case 3: If S1[i] is neither ‘?’ nor ‘*’
then we can say as the characters at i and j don’t match then the strings don’t match, so we return false.
Step 3: Return logical OR (||) of all the ChoicesBase Cases We are reducing i and j in our recursive relation, there can be two possibilities, either i becomes -1 or j becomes -1., i,e we exhaust either S1 or S2 respectively.
1. When S1 is exhausted:
When S1 is exhausted (i < 0), we know that in order for the strings to match, String S2 should also exhaust simultaneously. If it does, we return true, else we return false.
2. When S2 is exhausted:
When S2 is exhausted(j < 0) and S1 has not, there is only one pattern that can account for true(matching of strings). It is if S1 is like this “*”,”****”,”***”, i.e: S1 contains only stars. Then we can replace every star with a sequence of length 0 and say that the string match.
If S1 is all-stars, we return true, else return false.
The final pseudocode after steps 1, and 2
.png)
.png)
Sb Mai He Kru ...
Khud Bhi Kr le Khuch ..... Nalayk
Time & Space Complexity
Time Complexity: O(2^N)Reason: Exponential Time we find out the all the Possible Path
Space Complexity: O(N)
Reason: We are using a recursion stack space(O(N))
Memoization Approch
If we Observe in the recursion tree, we will observe a many number of overlapping subproblems. Therefore the recursive solution can be memoized for to reduce the time complexity.
Steps to convert Recursive code to memoization solution:.png)
.png)
Sb Mai He Kru ...
Khud Bhi Kr le Khuch ..... Nalayk
Time & Space Complexity
Time Complexity:O(N*M)Reason: There are N*M states therefore at max ‘N*M’ new problems will be solved.
Space Complexity: O(N*M) + O(N+M)
Reason: We are using a recursion stack space(O(N+M)) and a 2D array ( O(N*M)).
Tabulation Approch
Tabulation is a ‘bottom-up’ approach where we start from the base case and reach the final answer that we want and Memoization is the Top-down Approch.In Tabulation Approch We Just Creat a DP Array Same as Memoization and Simply Convert the Recurance Relation into the form of the Looping
VVVV Important Point: Tabulation Approch is Always 1-Based Indexing Always RememberSteps to convert Recursive Solution to Tabulation one.
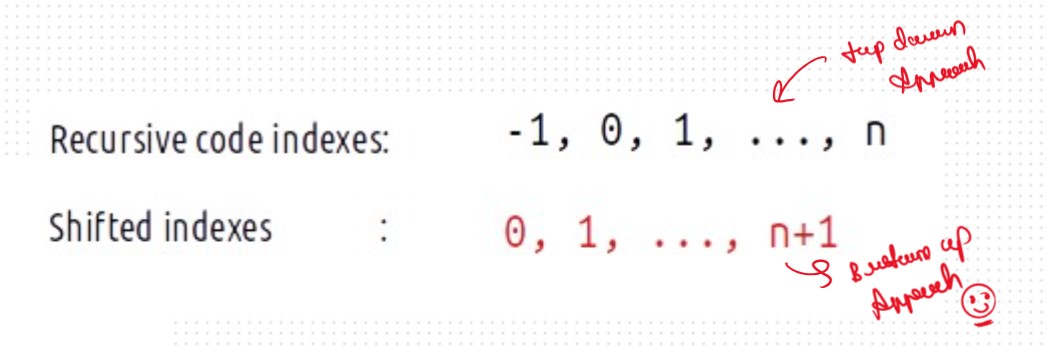
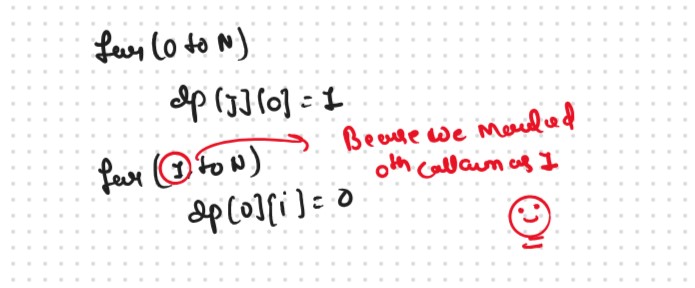
.png)
.png)
Sb Mai He Kru ...
Khud Bhi Kr le Khuch ..... Nalayk
Time & Space Complexity
Time Complexity: O(N*M)Reason:There are 2 nested loops
Space Complexity: O(N*M)
Reason: We are using an external array of size ‘N*M’. Stack Space is eliminated.
Space Optimization
If we closelly Observed if any Tabulation Approch we used the Some Limited Stuff like: dp[i][j] = dp[i-1][j-1], dp[i][j] = dp[i-1][j] ||dp[i][j-1] for the finding the our ans then definetly here Spaced Optimization is Possible in that types of Problems. Always Remember
Golden Rule
.png)
.png)
Sb Mai He Kru ...
Khud Bhi Kr le Khuch ..... Nalayk
Time & Space Complexity
Time Complexity: O(N*M)Reason: There are three 2 nested loops
Space Complexity: O(W)
Reason: We are using an external array of size ‘M+1’ to store only one row.