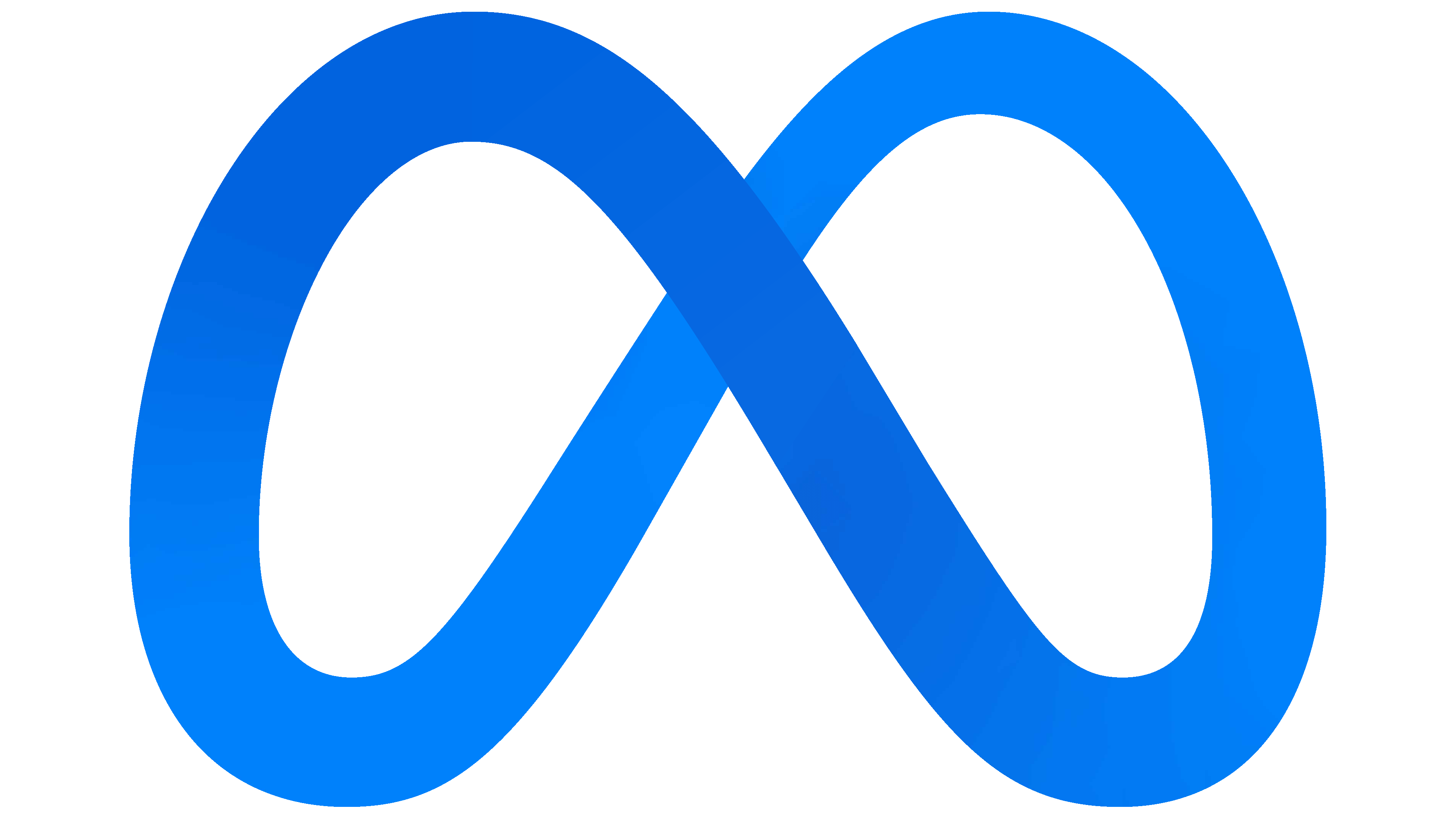

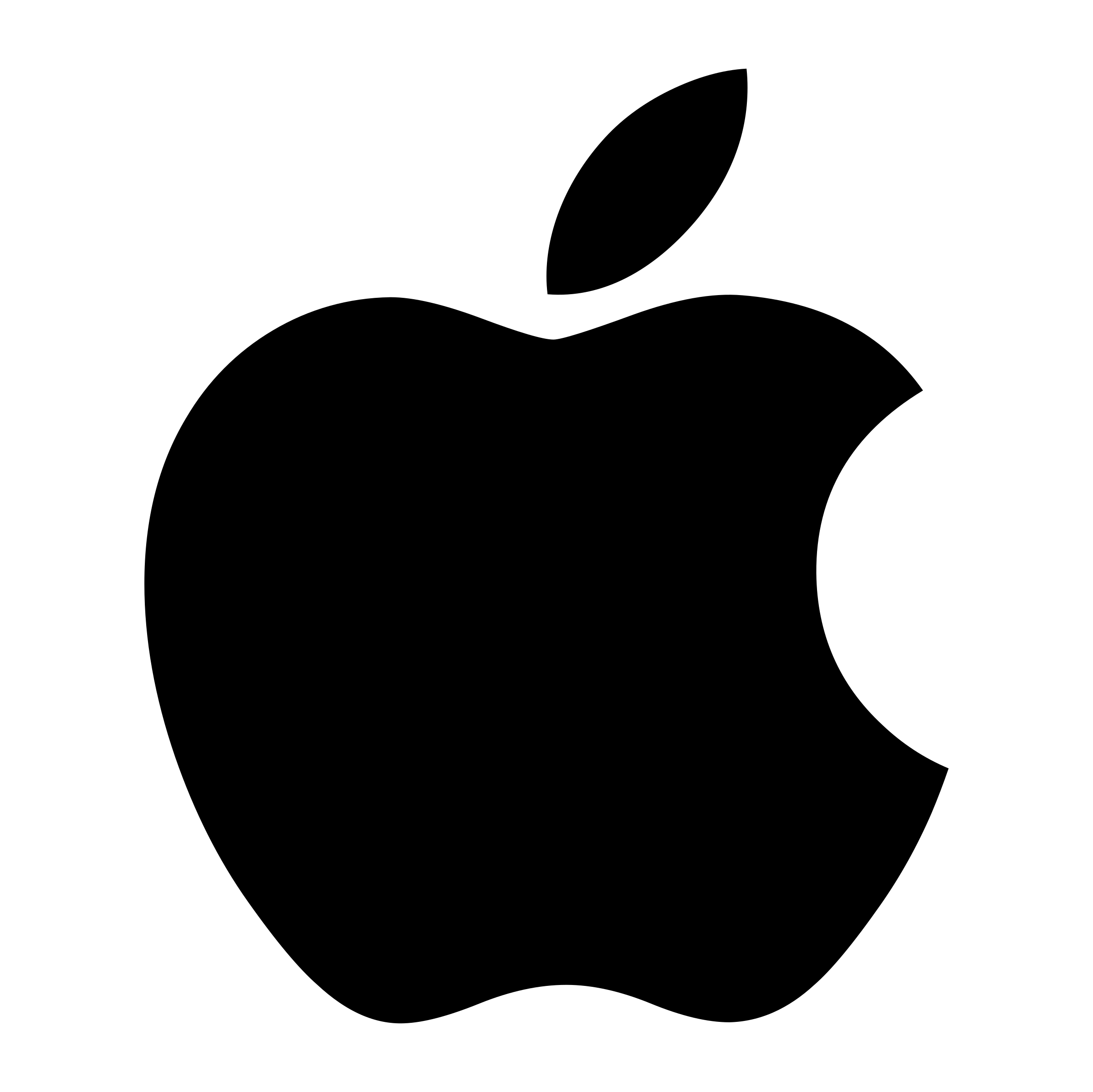
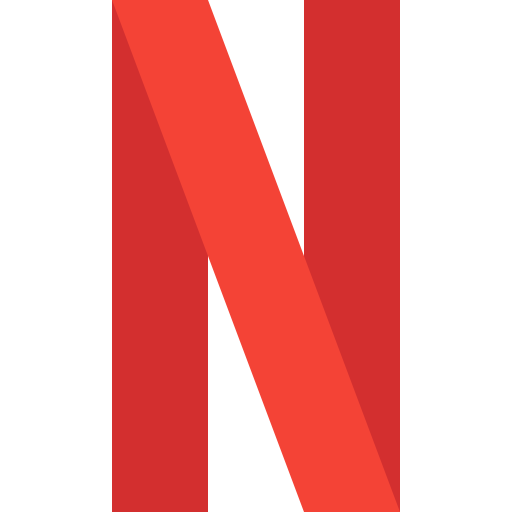
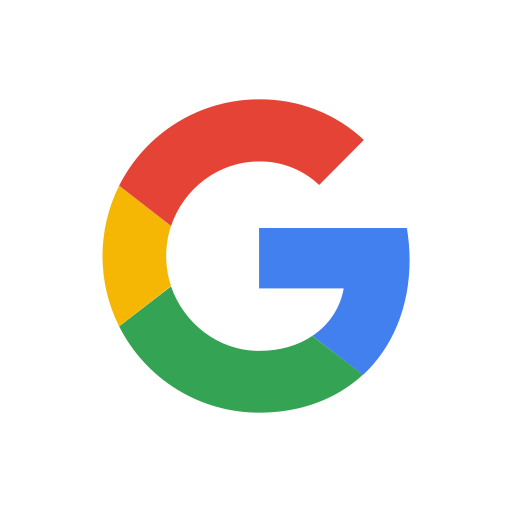
Jb Tak Fodega Nhi 🎯 Tb Tk Chodega Nhi 📚 (MAANG)
DPL35 Best Time to Buy and Sell Stock I
Best time to buy and sell stock I, We are given an array Arr[] of length n. It represents the price of
a stock on ‘n’ days. The following guidelines need to be followed:
How To Solved this Problem
For every index of string S1, we have different options to match that index with string S2. Therefore,
we can think in terms of string matching path as we have done already in previous questions. Again
this Problem Based on the DP on String Matching
VVVV Important Note:
Intuition Behid this Problem
We need to find the maximum profit. In order to maximize the profit, we need to buy the stock at its
lowest price and sell it when the price is highest. In the given example the lowest price(1) is on Day
1 and the highest price is (7) is on Day 0. But we need to buy the stock before selling it therefore
we need to modify this approach.
We can try to sell the stock every day and try to find the maximum profit that can be generated by
selling the stock on that day. At last, we can return the maximum of these individual maximum profits
as our answer.
To find the individual maximum profit on a day ‘i’, we know the selling price, i.e the price of the
stock on day i, we need to find the buying price. To maximize the profit on day ‘i’, the buying price
should be the lowest price of the stock from day 0 to day i (see the graph above). This way we can
keep track of the correct buying price for any day.
Approch
The algorithm approach can be stated as:
VVVV Important Note:
This Problem already take the liner time to solved this probelm and Space complexcity is Also take the
Constant, so its not possible to more Optimized this Solution that's why we Direct the Optimial Code.
But in the Upcomming Problems on DP on Stock we will used the Same Approches and Flow that we used on
the Previous Patterns on DP
class Solution: def maxProfit(self, prices): maxProfit = 0 minPrice = prices[0] for index in range(1,len(prices)): currentStockPrice = prices[index] - minPrice maxProfit = max(maxProfit,currentStockPrice) minPrice = min(minPrice,prices[index]) print(minPrice) return maxProfit ans = Solution() prices = [7,1,5,3,6,4] print(ans.maxProfit(prices))
Time & Space Complexity
Time Complexity: O(N)Reason: We are performing a single iteration
Space Complexity: O(1)
Reason: No extra space is used. Just we used the Some Variables for the Storing the Some Dummy Data