JB TAK FODEGA NHI .... TB TK CHODEGA NHI .... (MAANG)
SQP11 Largest Rectangle in Histogram
Given an array of integers heights representing the histogram's bar height where the width of each bar is 1, return the area of the largest rectangle in the histogram.
Example 1:
Input:heights = [2,1,5,6,2,3]
Output: 10
Explanation:
The above is a histogram where width of each bar is 1.
The largest rectangle is shown in the red area, which has an area = 10 units.
Constraints:
- 1 <= heights.length <= 10^5
- 0 <= heights[i] <= 10^4
Notes
Note: Zoom for Better Understanding
Brute Force Approach
Intuition: The intuition behind the approach is taking different bars and finding the maximum width possible using the bar.Always try to Strat your Interview with the Brute Force Approach and then go with the Optimal Solution
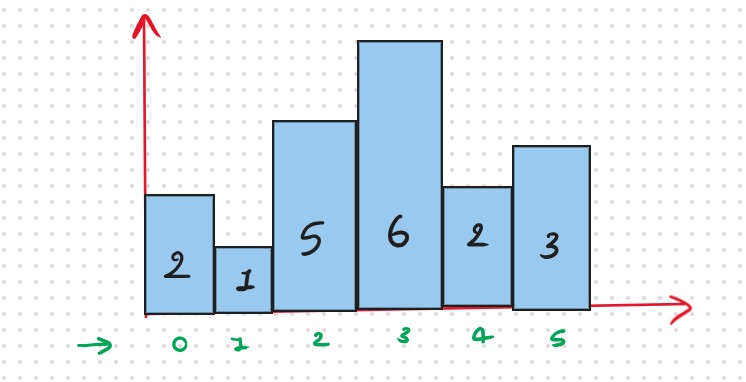
All Areas
Now the Question is How to find out the Left and Right all the Rectangualar box that Comes under the particular Rectangular box
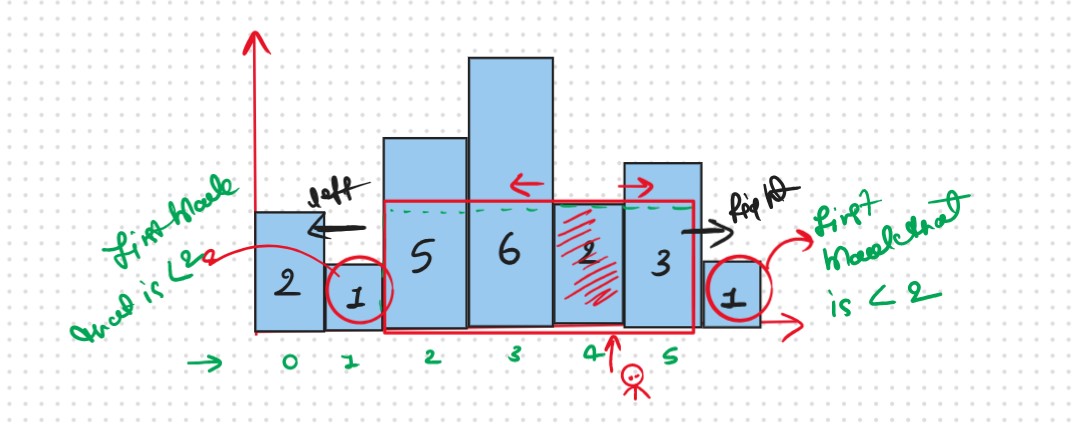
Assume that we are Standing on the Box = 2, Index = 4 finding the all box that are comes under tha Range
Formula: last Index and first Index of the first Smaller box for the particular box + 1
count = LastIndex-FirstIndex + 1
ans = count * arr[i]
Example:
Box = 2
firstIndex = 2
lastIndex = 5
count = 5-2 + 1
ans = 4 * 2
ans = 8
Code Zone!
Before Loking the Code...
Try by Your Self ...
.png)
.png)
Sb Mai He Kru ...
Khud Bhi Kr le Khuch ..... Nalayk
Time Complexity:O(N*N) Nexted Iteration for the left and Rigth Index Finding and calculate the max Area
Space Complexity:O(1) Constant Space.
Optimized Approch
During the BruteForce Approch we finding the formula for finding the left and right index thatt is (LastIndex-FirstIndex) * arr[i] + 1
Prevesoly Smaller Element
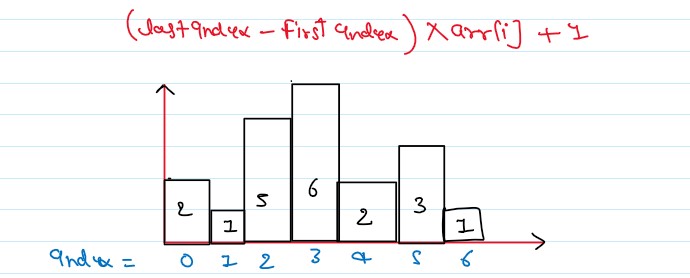
Let's Understand
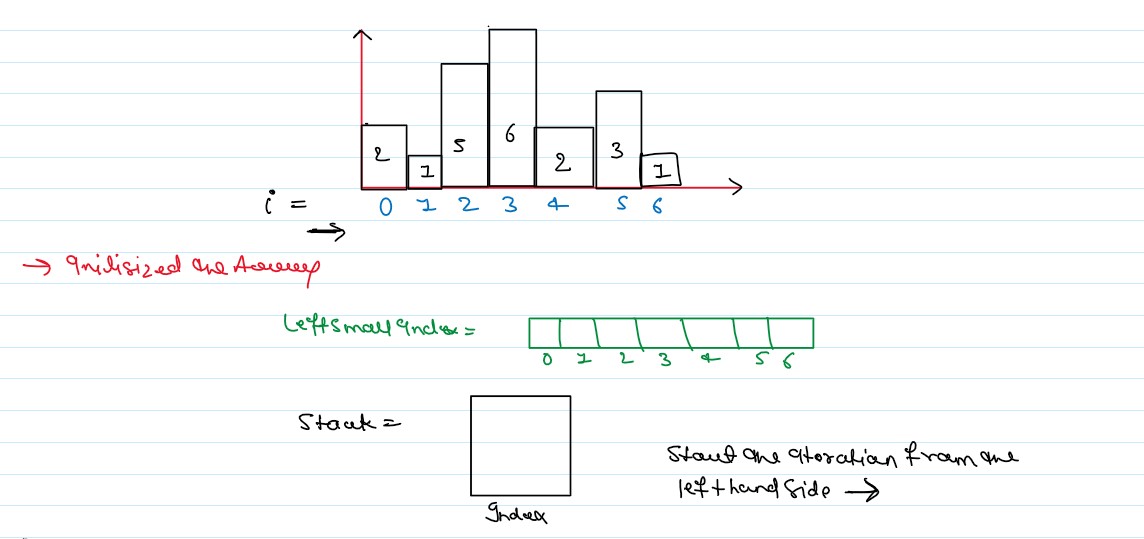
Inilisized the array with the empty stack and start iteation from -> side
Steps
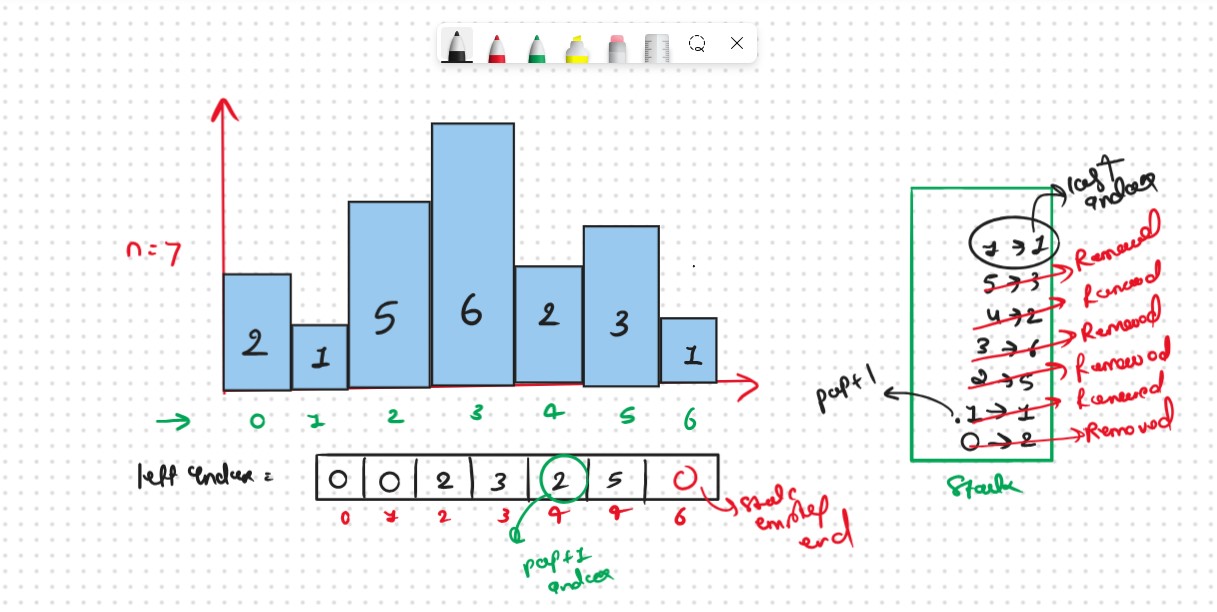

After finding the left adn rigtth small Index we iterate linearly and appy the formula that we find out (LastIndex-FirstIndex) * arr[i] + 1
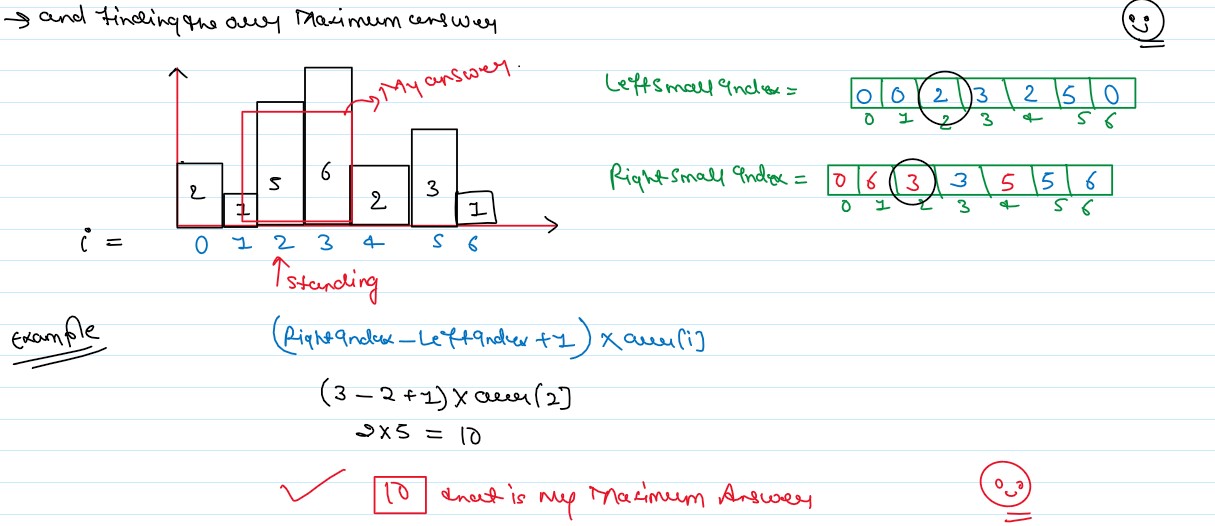
.png)
.png)
Sb Mai He Kru ...
Khud Bhi Kr le Khuch ..... Nalayk
Time Complexity:O(N) + O(N) + O(N) ~ O(N) for left array, for rigtth array and finding the Maximum ans
Space Complexity:O(N) + O(N) + O(N) ~ O(N) for left array, for rigtth array and Stack
Most Optimized Approch
As our Prevesoly approch we sued the formula that is (LastIndex-FirstIndex) * arr[i] + 1 that take 2 passes
Now in this Approch our task is Convert 2 Passes into the 1 Pass
Thought Process
.png)
.png)
Sb Mai He Kru ...
Khud Bhi Kr le Khuch ..... Nalayk
Time Complexity:O(N) + O(N) forf Linear Iteration and Maintain the Stack [ Every time we not Removed the Every element from the stack ]
Space Complexity:O(N) Only for the Stack No need to required another data structure.