JB TAK FODEGA NHI .... TB TK CHODEGA NHI .... (MAANG)
SQP13 Rotting Oranges
You are given an m x n grid where each cell can have one of three values:
Every minute, any fresh orange that is 4-directionally adjacent to a rotten orange becomes rotten.
Return the minimum number of minutes that must elapse until no cell has a fresh orange. If this is impossible, return -1.
Example 1:
Input: grid = [[2,1,1],[1,1,0],[0,1,1]]
Output: 4
Explanation:
The orange in the bottom left corner (row 2, column 0) is never rotten, because rotting only happens 4-directionally.
Example 2:
Input: grid = [[0,2]]
Output: 0
Explanation:
Since there are already no fresh oranges at minute 0, the answer is just 0.
Constraints:
- m == grid.length
- n == grid[i].length
- 1 <= m, n <= 10
- grid[i][j] is 0, 1, or 2.
Notes
Note: Zoom for Better Understanding
Thought Process
This Question Based on the Graph But we Implement in the Stack and Queue
In this Problem we have a M X N grid and we have a some Instruction.
Here we used the concept of the BFS and we required the Queue Data Structure
Basically we first count the fresh,rotten and empty cell in the Matrix
The algorithm steps are as follows:
Consider the following example to understand how BFS traverses the cells and rotten the oranges accordingly.
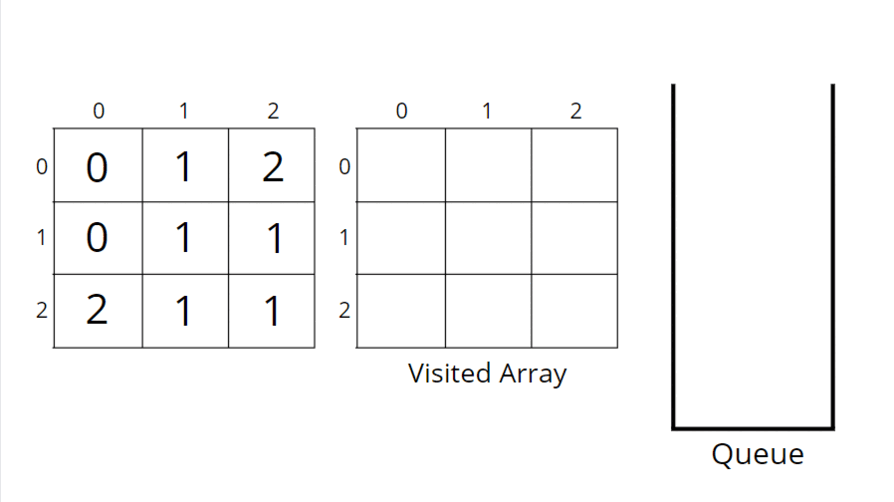
How do set boundaries for 4 directions?
The 4 neighbours will have the following indexes:
Now, either we can apply 4 conditions or follow the following method.
From the above image, it is clear that the delta change in a row is -1, +0, +1, +0. Similarly, the delta change in column is 0, +1, +0, -1. So we can apply the same logic to find the neighbours of a particular pixel (row, column).
Code Zone!
.png)
.png)
Sb Mai He Kru ...
Khud Bhi Kr le Khuch ..... Nalayk
Time Complexity:O(NxM + NxMx4) ~ O(N x M) For the worst case, all of the cells will have fresh oranges, so the BFS function will be called for (N x M) nodes and for every node, we are traversing for 4 neighbours, it will take O(N x M x 4) time.
Space Complexity:O(N x M), O(N x M) for copied input array and recursive stack space takes up N x M locations at max.